Python is a great programming language due to a multitude of reasons such as a long list of libraries ready to solve almost any problem you might encounter. For me, however, the biggest reason to prefer coding in Python with Unity over C# is that I simply don't have to type as much; no type declarations, semicolons or curly brackets, not to mention return types, scopes and so on… 😴
Yet, online I can only find outdated guides or worse yet "why don't you just learn C#, it's easy and blah blah" comments. 😫 But I like Python. 🥺Therefore, this post teaches you how to use IronPython with Unity. Just remember that IronPython only supports Python 2.
Use Python with Unity
First you will have to download IronPython dll files and place them into Assets/Plugins directory. The easiest way to do this is by downloading my Unity package.
Import my Unity package to your game project
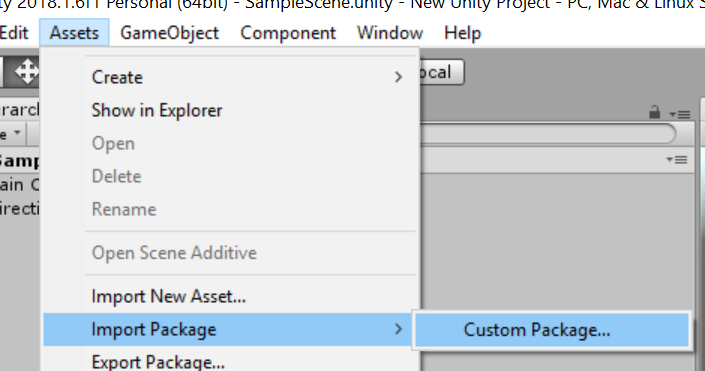
IronPython requires .NET 4.5, and Unity doesn't like this by default. That's why you will have to change the .NET version by going to Edit -> Project Settings -> Player. Make sure that the Scripting Runtime Version is .NET 4.x (this requires you to restart Unity) and that API Compatibility level is .NET 4.x
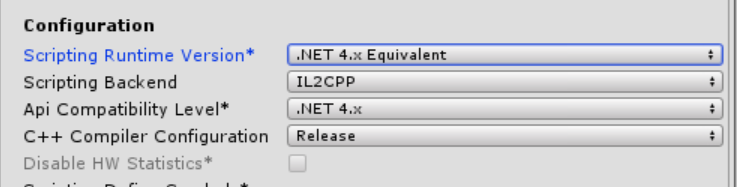
How to code in Python
Assume you have a small code snippet greeter.py in Python like this:
class Greeter(): def __init__(self, name): self.name = name def greet(self): return "Hi, " + self.name
You can use it from C# like this
using System.Collections; using System.Collections.Generic; using IronPython.Hosting; using UnityEngine; public class PythonInterfacer : MonoBehaviour { void Start () { var engine = Python.CreateEngine(); ICollection<string> searchPaths = engine.GetSearchPaths(); //Path to the folder of greeter.py searchPaths.Add(@"C:\Users\Mika\Documents\MyPythonScripts\"); //Path to the Python standard library searchPaths.Add(@"C:\Users\Mika\Documents\MyUnityGame\Assets\Plugins\Lib\"); engine.SetSearchPaths(searchPaths); dynamic py = engine.ExecuteFile(@"C:\Users\Mika\Documents\MyPythonScripts\greeter.py"); dynamic greeter = py.Greeter("Mika"); Debug.Log(greeter.greet()); } }
For a similar example, see the PythonExample prefab in my Unity package.
As you can see, the Python class can be directly used as an object in C#, and better yet, if you provide the path to the Assets\Plugins\Lib folder included in my Unity package, you can use anything in Python standard library! In addition, if you also provide the path to your Python script, you can import anything in that path as usual.
Next steps
If you want to use any external Python libraries (the ones you install with pip), you can just download and extract them to the
Assets\Plugins\Lib folder. This will make them available for IronPython.
Note: Using absolute paths is fine when you are doing development, but if you ever decide to publish your game, you will need to resolve the paths dynamically and distribute the standard library in Assets\Plugins\Lib and your Python scripts with the game executable.
Finally you can have the best of two worlds: Unity's dead easy world editor and Pythonic simplicity. ☺️